Pandas, a highly popular library in the Python ecosystem, is renowned for its robust data manipulation capabilities. One of its most powerful features is the .iloc indexer, which allows users to select rows and columns by integer-location based indexing. This method is particularly useful when you need to access data by its numerical position rather than its label or name. Whether you're cleaning a dataset, performing exploratory data analysis, or transforming data for machine learning models, .iloc is an indispensable tool in your data manipulation toolkit. As we delve deeper into the functionality of .iloc in Pandas, this guide will provide a comprehensive understanding of how to use it effectively. We will explore its syntax, applications, and best practices, ensuring you are well-equipped to leverage this powerful feature in your data projects. From the basics to advanced applications, this article aims to cover all aspects of .iloc, making it a valuable resource for both beginners and experienced data professionals.
Table of Contents
- Understanding Pandas and DataFrames
- Introduction to .iloc
- Basic Usage of .iloc
- Selecting Rows with .iloc
- Selecting Columns with .iloc
- Slicing with .iloc
- Advanced .iloc Techniques
- Common Mistakes and How to Avoid Them
- Performance Considerations
- Comparison with Other Indexers
- Case Study: Applying .iloc in Real-World Data
- Frequently Asked Questions
- Conclusion
Understanding Pandas and DataFrames
Pandas is an open-source data analysis and manipulation tool built on top of the Python programming language. It is widely used for data wrangling, cleaning, and preparation, offering data structures and operations for manipulating numerical tables and time series data. One of the fundamental data structures in Pandas is the DataFrame, which resembles a table and allows for data manipulation with ease.
A DataFrame in Pandas is a two-dimensional, size-mutable, potentially heterogeneous tabular data structure with labeled axes (rows and columns). It is similar to a SQL table or a spreadsheet data format. DataFrames allow you to store and manipulate large datasets efficiently, making them a cornerstone of data analysis workflows.
The power of Pandas lies in its ability to handle a diverse range of data types and sources, from CSV files and Excel spreadsheets to SQL databases and web data. With Pandas, data manipulation tasks that would require complex SQL queries or multiple lines of code in other languages can often be accomplished with a single line of Python code.
Introduction to .iloc
The .iloc indexer in Pandas is used for selecting data based on its integer location. This means you can access rows and columns by their numerical index, which is particularly useful when dealing with datasets where labels are not available or when you want to perform operations based on position.
.iloc stands for 'integer location' and is part of a suite of indexers in Pandas, including .loc and .at. While .loc allows for label-based indexing, .iloc is strictly integer-based, providing a powerful way to slice and dice your data by position.
The syntax for .iloc is straightforward. It uses the following format: DataFrame.iloc[row_index, column_index]
. Here, row_index
and column_index
can be single integers, slices, or lists of integers, allowing for flexible data selection.
Basic Usage of .iloc
Mastering the basic usage of .iloc is essential for effective data analysis with Pandas. At its core, .iloc enables you to select data from a DataFrame using integer-based indexing. This is particularly helpful when you need to work with numerical positions rather than labels.
For example, if you have a DataFrame named df
and you want to select the first row, you would use the following command: df.iloc[0]
. Similarly, to select the first column, you would use: df.iloc[:, 0]
. Here, the colon (:) is used to denote all rows or columns, depending on its position.
One of the key advantages of .iloc is its ability to select multiple rows and columns simultaneously. You can achieve this by passing slices or lists of integers. For instance, to select the first two rows and the first three columns, you would use: df.iloc[0:2, 0:3]
. This flexibility makes .iloc a powerful tool for data manipulation and extraction.
Selecting Rows with .iloc
Selecting rows using .iloc is a common task in data analysis. Whether you want to access a single row or multiple rows, .iloc provides an efficient way to do so using integer-based indexing.
To select a single row, simply pass the row index to .iloc. For example, df.iloc[3]
selects the fourth row in the DataFrame. Remember, Python uses zero-based indexing, so the index starts from zero.
If you need to select multiple rows, you can use a slice or a list of indices. For instance, df.iloc[1:4]
selects rows from the second to the fourth (excluding the fourth), and df.iloc[[0, 2, 5]]
selects the first, third, and sixth rows. This flexibility allows you to extract specific subsets of your data based on their position.
Selecting Columns with .iloc
Just as with rows, selecting columns with .iloc is straightforward and follows a similar syntax. By specifying the column index or indices, you can extract the desired columns from a DataFrame.
To select a single column, use the following syntax: df.iloc[:, 2]
. This selects the third column from the DataFrame, with the colon indicating all rows. If you want to select multiple columns, you can use slices or lists. For example, df.iloc[:, 1:4]
selects the second to fourth columns, while df.iloc[:, [0, 3, 5]]
selects the first, fourth, and sixth columns.
Using .iloc to select columns is particularly useful when you need to isolate specific variables for analysis or when you want to create new DataFrames with selected features.
Slicing with .iloc
Slicing is one of the most powerful features of .iloc, allowing you to extract specific parts of your DataFrame with ease. By combining row and column indices, you can create subsets of your data for more focused analysis.
The syntax for slicing with .iloc involves using the colon (:) to denote ranges. For example, df.iloc[0:5, 1:3]
selects rows 0 to 4 and columns 1 to 2. This creates a smaller DataFrame containing only the specified rows and columns.
Slicing is particularly useful when working with large datasets, as it allows you to focus on relevant sections of the data without loading the entire DataFrame into memory. This can significantly improve performance and make your analysis more efficient.
Advanced .iloc Techniques
Beyond basic selection and slicing, .iloc offers advanced techniques for more sophisticated data manipulation. These techniques allow you to perform complex operations and extract data with precision.
One advanced technique involves using Boolean arrays with .iloc. By creating a Boolean array that represents the condition you want to apply, you can pass it to .iloc to select rows or columns that meet the criteria. For example, if you have a condition array named condition
, you can select rows that meet the condition using df.iloc[condition]
.
Another technique is using .iloc with numpy functions. Since .iloc works well with numerical indexing, you can combine it with numpy functions for operations such as reshaping, transposing, or applying mathematical operations across selected data.
Common Mistakes and How to Avoid Them
While .iloc is a powerful tool, it is not without its pitfalls. Understanding common mistakes and how to avoid them is crucial for using .iloc effectively.
One common mistake is confusing .iloc with .loc. Remember, .iloc is based on integer indexing, while .loc is label-based. Using the wrong indexer can lead to errors and unexpected results.
Another mistake is using out-of-bounds indices. Since .iloc expects integer indices, providing an index that exceeds the DataFrame's dimensions will raise an IndexError. Always verify the shape of your DataFrame before performing .iloc operations.
Lastly, be cautious of modifying data in place. While .iloc allows for direct data manipulation, it's often safer to work with copies of your data to prevent unintentional modifications.
Performance Considerations
When working with large datasets, performance considerations become critical. Although .iloc is efficient, understanding its performance implications can help optimize your data workflows.
One performance consideration is the computational cost of indexing operations. While .iloc is generally fast, repeatedly accessing large portions of data can slow down your analysis. Whenever possible, try to minimize the number of indexing operations and work with smaller subsets of data.
Another consideration is memory usage. While .iloc does not inherently increase memory usage, creating many intermediate DataFrames through slicing can lead to increased memory consumption. Be mindful of memory usage, especially when working with limited resources.
Comparison with Other Indexers
Pandas offers several indexers, each with its unique features and use cases. Understanding the differences between .iloc and other indexers like .loc and .at can help you choose the right tool for your task.
.iloc is best suited for integer-based indexing, allowing you to access data by its numerical position. In contrast, .loc is label-based and allows you to access data by its labels or names. This makes .loc more intuitive when working with labeled datasets.
.at is another indexer designed for fast access to scalar values. It's similar to .iloc but optimized for single element access, making it ideal for tasks that require frequent access to individual elements.
Case Study: Applying .iloc in Real-World Data
To illustrate the practical application of .iloc, let's explore a case study involving real-world data. Suppose you have a dataset containing information about sales transactions, and you want to analyze sales trends over time.
Initially, you might use .iloc to select specific columns of interest, such as transaction dates and sales amounts. By slicing the DataFrame with .iloc, you can create a new DataFrame containing only the relevant data for analysis.
Next, you could use .iloc to filter rows based on specific time periods or sales regions. For example, by combining .iloc with numpy functions, you can extract data for a particular year or region, allowing for more focused analysis.
Finally, you could apply advanced .iloc techniques to perform detailed analysis. For instance, by using Boolean arrays with .iloc, you can identify trends or anomalies in sales data, helping you make informed business decisions.
Frequently Asked Questions
What is the primary purpose of .iloc in Pandas?
The primary purpose of .iloc in Pandas is to provide a way to select and manipulate data based on its integer position within a DataFrame. It allows you to access rows and columns by their numerical index, offering flexibility and precision in data manipulation tasks.
How does .iloc differ from .loc in Pandas?
.iloc differs from .loc in that it uses integer-based indexing, while .loc is label-based. This means .iloc accesses data by its numerical position, whereas .loc accesses data by its labels or names. Understanding this distinction is crucial for selecting the appropriate indexer for your task.
Can .iloc be used with negative indices?
Yes, .iloc can be used with negative indices, which allows you to access data from the end of the DataFrame. For example, df.iloc[-1]
selects the last row of the DataFrame, and df.iloc[:, -2]
selects the second-to-last column.
Is it possible to modify data using .iloc?
Yes, .iloc allows for data modification. You can directly assign new values to selected rows or columns using .iloc. However, it's important to be cautious when modifying data in place, as it can lead to unintentional changes in your dataset.
What are some common use cases for .iloc?
Common use cases for .iloc include data cleaning, data extraction, and feature selection. It is often used in scenarios where you need to access or manipulate data by its position, such as selecting specific rows and columns for analysis or creating new DataFrames with selected features.
How does .iloc handle out-of-bounds indices?
If you provide an out-of-bounds index to .iloc, it will raise an IndexError. It's important to verify the dimensions of your DataFrame before using .iloc to ensure that the indices you provide are within the valid range.
Conclusion
Understanding and effectively using .iloc in Pandas is essential for anyone working with data in Python. Its flexibility and precision make it a powerful tool for data manipulation, allowing you to access and modify data based on its integer position within a DataFrame. By mastering .iloc, you can enhance your data analysis workflows and gain deeper insights from your datasets. Whether you're a beginner or an experienced data professional, this guide provides the knowledge and techniques you need to leverage .iloc to its fullest potential.
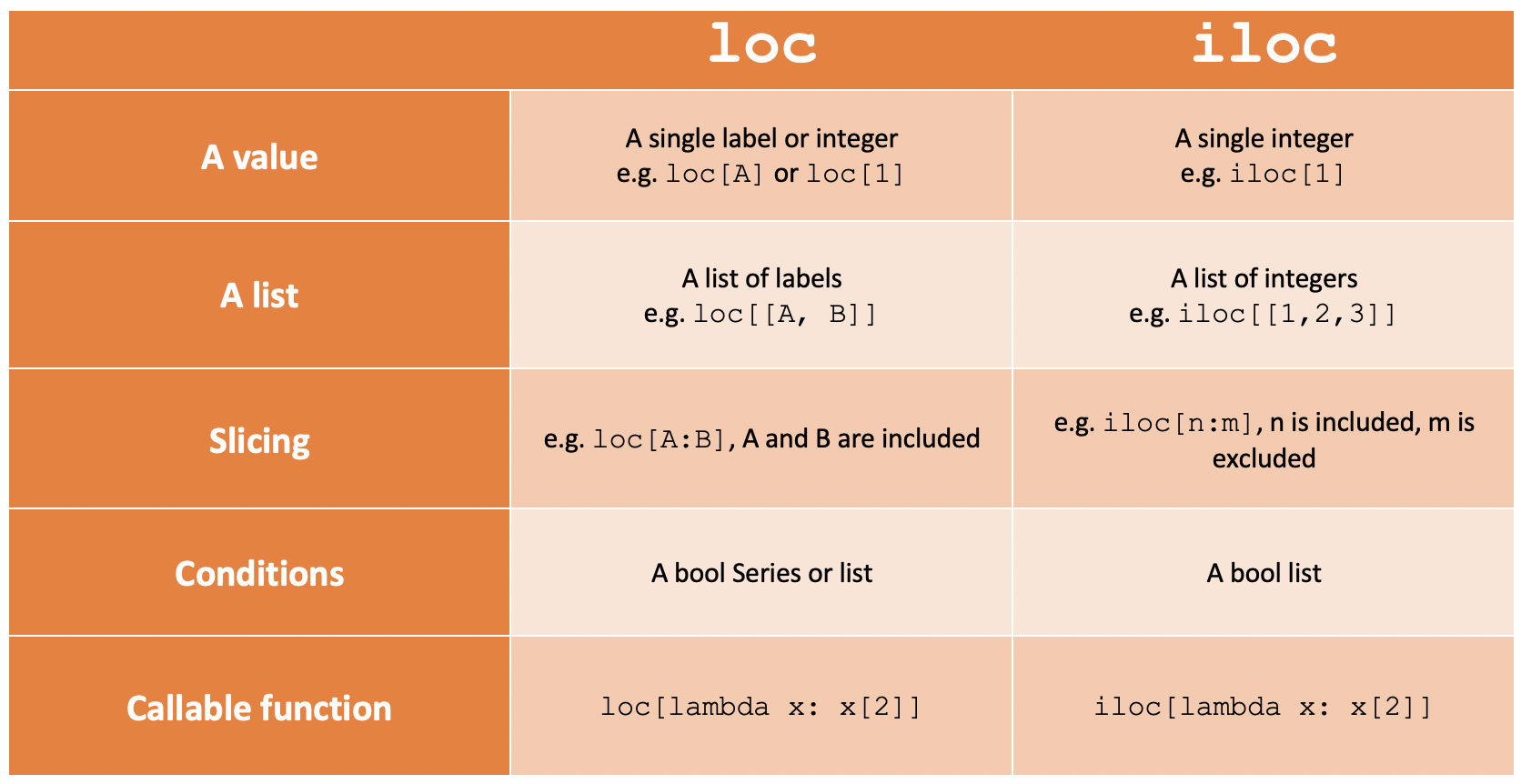
